チェックボックスの値を判定する
DataGridViewで選択した行のチェックボックスの値を判定する方法です。
Public Class Form1
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
'ユーザーからのデータ追加を不可にしておく
DataGridView1.AllowUserToAddRows = False
'CheckBox列を追加する
Dim column As New DataGridViewCheckBoxColumn
DataGridView1.Columns.Add(column)
'列数設定
DataGridView1.ColumnCount = 4
DataGridView1.RowCount = 4
'列名設定
DataGridView1.Columns(0).HeaderText = ""
DataGridView1.Columns(1).HeaderText = "商品名"
DataGridView1.Columns(2).HeaderText = "製造元"
DataGridView1.Columns(3).HeaderText = "価格"
DataGridView1.Rows(0).Cells(0).Value = True
DataGridView1.Rows(0).Cells(1).Value = "商品A"
DataGridView1.Rows(0).Cells(2).Value = "製造元A"
DataGridView1.Rows(0).Cells(3).Value = "1000"
DataGridView1.Rows(1).Cells(0).Value = False
DataGridView1.Rows(1).Cells(1).Value = "商品B"
DataGridView1.Rows(1).Cells(2).Value = "製造元B"
DataGridView1.Rows(1).Cells(3).Value = "3000"
DataGridView1.Rows(2).Cells(0).Value = True
DataGridView1.Rows(2).Cells(1).Value = "商品C"
DataGridView1.Rows(2).Cells(2).Value = "製造元C"
DataGridView1.Rows(2).Cells(3).Value = "5000"
DataGridView1.Rows(3).Cells(0).Value = False
DataGridView1.Rows(3).Cells(1).Value = "商品D"
DataGridView1.Rows(3).Cells(2).Value = "製造元D"
DataGridView1.Rows(3).Cells(3).Value = "10000"
End Sub
Private Sub Button1_Click_1(sender As Object, e As EventArgs) Handles Button1.Click
Dim str As String
'選択している行の行番号の取得
Dim i As Integer = DataGridView1.SelectedCells(0).RowIndex
'選択している行の各項目を取得
str = DataGridView1.Rows(i).Cells(0).Value
str &= vbCrLf
str &= DataGridView1.Rows(i).Cells(1).Value
str &= vbCrLf
str &= DataGridView1.Rows(i).Cells(2).Value
str &= vbCrLf
str &= DataGridView1.Rows(i).Cells(3).Value
Label1.Text = str
End Sub
End Class
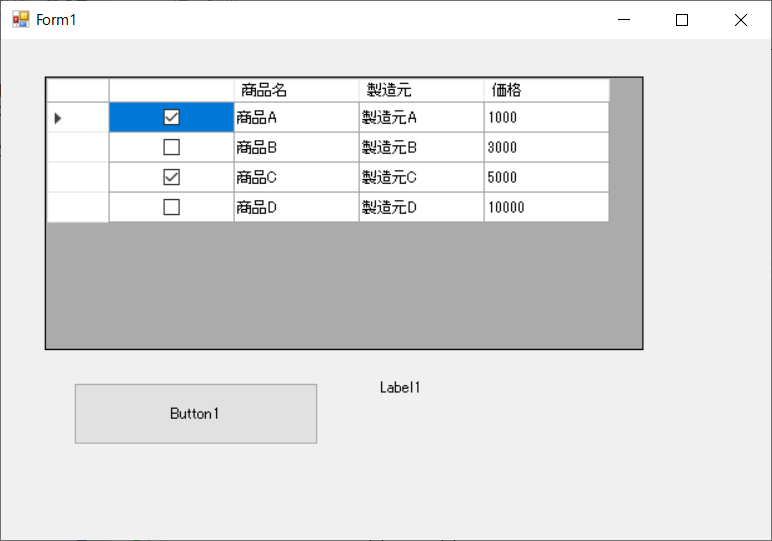
1列目にチェックボックスを配置しました。
ボタンを押すと、
選択したセルの行のチェックボックスの値と
他のデータをラベルに表示するプログラムです。
選択されているセルの情報を得るには、SelectedCells(0)プロパティを使います。
そして、チェックボックスは1列目なので、
DataGridView1.Rows(行番号).Cells(0).Valueの値で取得できます。
チェックされている状態なら「True」が返ります。(厳密には0以外の数値)
チェックされていない状態なら「False」が返ります。(厳密には数値の0)
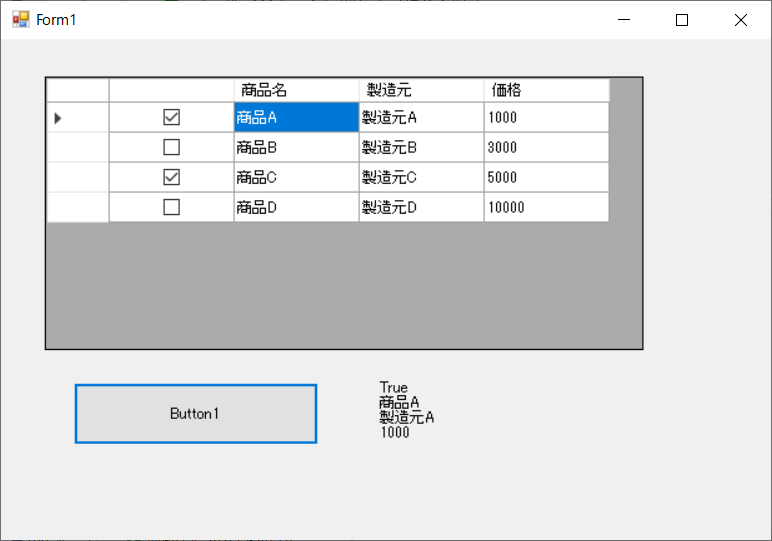
1行目を選択した結果が上記です。
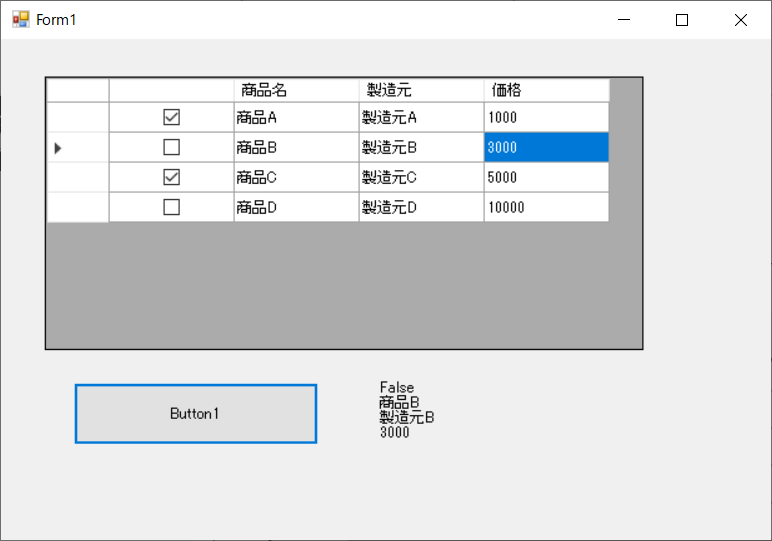
2行目を選択した結果が上記です。
チェックボックスの値
チェックボックスの値に設定できる値は、
TrueかFalseのほかに、数値であれば、自由に指定する事ができます。
(他の設定によってはエラーが出るかも知れません。)
0ならば、チェックオフの状態になり、
0以外の数値ならばチェックされている状態になります。
チェックボックスのオン、オフを判定する時は
基本的には「Trueの場合」か「Falseの場合」で
判定して良いと思いますが、
期待通りの結果が得られない場合には、
「0の場合」と「0以外の場合」で判定してみて下さい。
また、プログラム実行後、
ユーザーからマウスでチェックのオン、オフを行った時は、
オンにすると、Trueの値が入力され、
オフにすると、Falseの値が入力されます。
これで、チェックボックスの値を判定する事ができるようになりました。