DataGridViewで選択した行の値を取得する方法です。
Public Class Form1
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
'ユーザーからのデータ追加を不可にしておく
DataGridView1.AllowUserToAddRows = False
'列数,行数設定
DataGridView1.ColumnCount = 4
DataGridView1.RowCount = 4
'列名設定
DataGridView1.Columns(0).HeaderText = "No"
DataGridView1.Columns(1).HeaderText = "商品名"
DataGridView1.Columns(2).HeaderText = "製造元"
DataGridView1.Columns(3).HeaderText = "価格"
'値をセット
DataGridView1.Rows(0).Cells(0).Value = 1
DataGridView1.Rows(0).Cells(1).Value = "商品A"
DataGridView1.Rows(0).Cells(2).Value = "製造元A"
DataGridView1.Rows(0).Cells(3).Value = 1000
DataGridView1.Rows(1).Cells(0).Value = 2
DataGridView1.Rows(1).Cells(1).Value = "商品B"
DataGridView1.Rows(1).Cells(2).Value = "製造元B"
DataGridView1.Rows(1).Cells(3).Value = 3000
DataGridView1.Rows(2).Cells(0).Value = 3
DataGridView1.Rows(2).Cells(1).Value = "商品C"
DataGridView1.Rows(2).Cells(2).Value = "製造元C"
DataGridView1.Rows(2).Cells(3).Value = 5000
DataGridView1.Rows(3).Cells(0).Value = 4
DataGridView1.Rows(3).Cells(1).Value = "商品D"
DataGridView1.Rows(3).Cells(2).Value = "製造元D"
DataGridView1.Rows(3).Cells(3).Value = 10000
End Sub
Private Sub Button1_Click_1(sender As Object, e As EventArgs) Handles Button1.Click
Dim str As String
'選択している行の行番号の取得
Dim i As Integer = DataGridView1.SelectedCells(0).RowIndex
'選択している行の各項目を取得
str = DataGridView1.Rows(i).Cells(0).Value
str &= vbCrLf
str &= DataGridView1.Rows(i).Cells(1).Value
str &= vbCrLf
str &= DataGridView1.Rows(i).Cells(2).Value
str &= vbCrLf
str &= DataGridView1.Rows(i).Cells(3).Value
'ラベルに表示
Label1.Text = str
End Sub
End Class
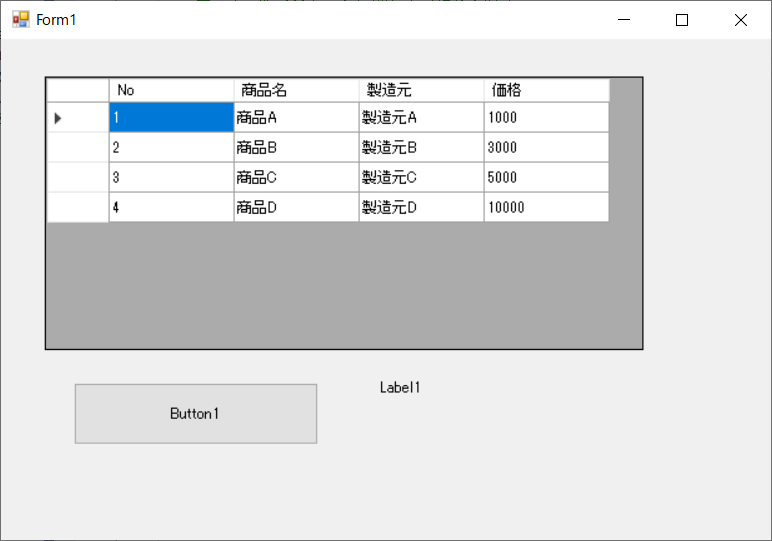
DataGridViewで好きなセルを選択し、ボタンを押すと
選択したセルの行の各項目をラベルに表示するプログラムです。
選択したセルの情報を取得するには、
SelectedCellsプロパティを使います。
このプログラムでは、1つのセル選択しか行いませんが、
マウスのドラッグやShiftキー、Ctrlキーを使って
複数のセルを選択する事ができます。
複数のセルを選択した場合にも、
SelectedCellsプロパティを使って、
選択した複数のセル1つ1つの情報にアクセスできます。
選択したセルが1つの時は、
SelectedCells(0) と書く事によって、
選択したセルの情報にアクセスする事ができます。
ここでは、選択したセルのRowIndex(行番号)を取得しています。
あとは、Rows Cells Value を使って、
データを取得して、ラベルに表示しています。
これで、選択した行のデータを取得する事ができるようになりました。
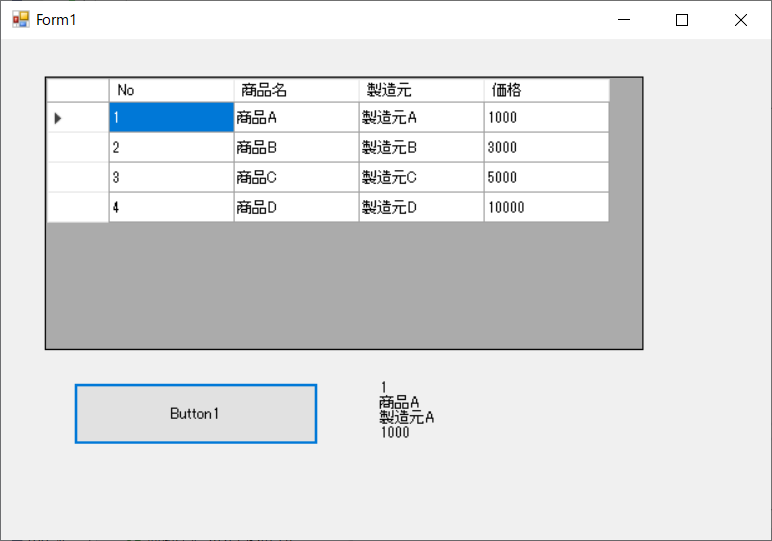
1行目を選択してボタンをクリックすると、ラベルに1行目のデータが表示されます。
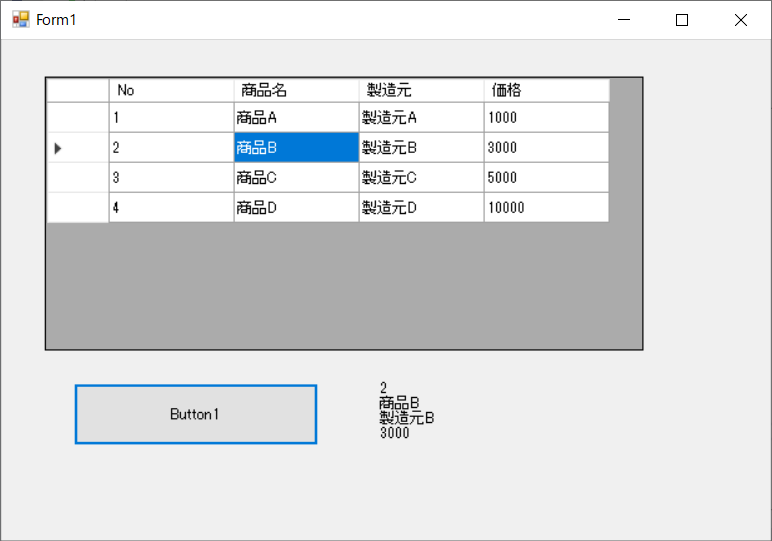
2行目の商品名を選択しても、2行目のデータを表示する事ができます。